Debounce and Throttle

From static web pages with flashy GIFs in the 90s to interactive, reactive modern web pages and applications. You have probably used or come across a website that uses search boxes with autocomplete, lists, areas with drag and drop functionality or similar things that require actively sending every change to the server that the user made and altering the UI depending on the response that comes from the server.
In this kind of scenario, to build a performant application, there are two techniques that are commonly used in well-known websites and applications which improve performance significantly. Thus, it becomes prevalent among modern web websites and applications.
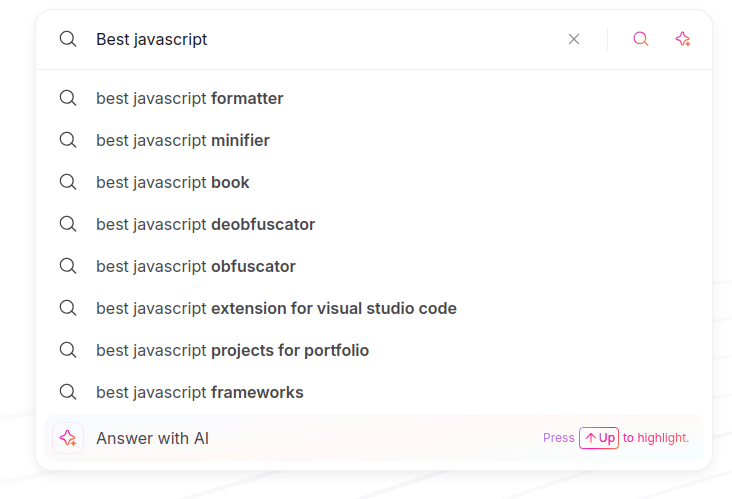
Debounce
The first technique I want to talk about is Debounce, this technique is the ideal solution for things like autocomplete text boxes such as search boxes that immediately show suggestions to the user depending on what user types.
Debounce works simply by delaying our function invocation for a certain period of time when a change happens in the textbox. If nothing happens during that period of time then our function will be invoked, and it sends a request to the server and retrieves the response then alters the UI. But if a change happens during that period of time, the countdown for delay will be restarted.
Let’s look at a simple example, in our example, let’s say we set a delay of 1 second which means our fetch request will only be called when the user has stopped for 1 second without interrupting and making changes in the input field. If the user types “best JavaScript” before stopping typing, a fetch request will be initiated after a 1 second delay and our function will return suggestions according to that input.
Let’s look at the implementation of debouncing,
Javascript:
function debounce(func, delay = 0) {
let timeoutId = null
return function (...args) {
// Keep a reference to 'this' so that func.apply or func.call can use it.
const thisRef = this
// Clear if there is a timeout already.
clearTimeout(timeoutId)
//Set new timeout
timeoutId = setTimeout(function () {
timeoutId = null // It's not strictly necessary but recommended to do this.
func.apply(thisRef, args) // alternatively, func.call(thisRef, ...args)
}, delay)
}
}
Typescript:
function debounce(func: Function, delay: number = 0): Function {
let timeoutId: ReturnType<typeof setTimeout> | null = null
return function (this: any, ...args: any[]) {
// Keep a reference to 'this' so that func.apply or func.call can use it.
const thisRef = this
// Clear if there is a timeout already.
clearTimeout(timeoutId ?? undefined)
//Set new timeout
timeoutId = setTimeout(function () {
timeoutId = null // It's not strictly necessary but recommended to do this.
func.apply(thisRef, args) // alternatively, func.call(thisRef, ...args)
}, delay)
}
}
Our debounce function accepts two parameters, the first one is the callback function and the second one is delay time. Inside the function, first, we create a reference ID for the setTimeout
function, and then we return a function that clears the previous timeout if exists and then creates a new timeout giving delay time.
After that, you may tempted to use immediately func(...args)
but this
value will be lost if the callback function is invoked that way. So, to preserve this
value, we use Function.prototype.apply()
or Function.prototype.call()
.
The main pitfall while implementing the proper debounce function is invoking the callback function with the correct this
value. Since the callback function will be invoked after the delay time, we need to ensure that the first argument to func.apply()
or func.call()
is the correct value. There are two ways we can achieve this:
- Using another variable like
thisRef
to keep a reference tothis
and accessthis
via that variable from within thesetTimeout
callback function. This way of preservingthis
value is considered a traditional way of preservingthis
value. After arrow functions are introduced with ES6, we don’t need to use an extra variable to preservethis
value in thesetTimeout
callback function.
JavaScript:
function debounce(func, delay = 0) {
let timeoutId = null
return function (...args) {
// Clear if there is a timeout already.
clearTimeout(timeoutId)
//Set new timeout
timeoutId = setTimeout(() => {
timeoutId = null // It's not strictly necessary but recommended to do this.
// Because of using arrow function, it carry over 'this' keyword of the outer function.
// So, we don't need to create reference to 'this' keyword of the outer function.
func.apply(this, args) // alternatively, func.call(this, ...args)
}, delay)
}
}
Typescript:
function debounce(func: Function, delay: number = 0): Function {
let timeoutId: ReturnType<typeof setTimeout> | null = null
return function (this: any, ...args: any[]) {
// Clear if there is a timeout already.
clearTimeout(timeoutId ?? undefined)
//Set new timeout
timeoutId = setTimeout(() => {
timeoutId = null // It's not strictly necessary but recommended to do this.
// Because of using arrow function, it carry over 'this' keyword of the outer function.
// So, we don't need to create reference to 'this' keyword of the outer function.
func.apply(this, args) // alternatively, func.call(this, ...args)
}, delay)
}
}
- Using an arrow function to declare the
setTimeout
callback function where thethis
value within it has lexical scope. Within an arrow function, the value ofthis
is bound to the context in which the function is created.
Another important thing is returned function shouldn’t be an arrow function because the this
value of the returned function needs to be dynamically determined when executed.
Throttle
Let’s now talk about the throttle technique, throttle is also used to limit the number of times a function is invoked, but the difference is the callback function is invoked immediately and doesn’t allow invocations again until delay time has passed.
A throttled function can be in one of the following states:
-
Idle: The throttled function was not invoked in the last
delay
time. When you call the throttled function, it will immediately execute the callback function without any need to throttle. After this, it enters to active state which we’ll talk about in a second. -
Active: The throttled function was invoked within the last
delay
time. Any call after this one shouldn’t execute the callback function untildelay
time is over.
Let’s look at the implementation of the throttle function:
JavaScript:
function throttle(func, delay = 0) {
let shouldThrottle = false
return function(...args){
if(shouldThrottle) return;
shouldThrottle = true // this will be true until delay time has passed.
setTimeout(function () {
shouldThrottle = false
}, delay)
func.apply(this, args) // alternatively, func.call(this, ...args)
}
}
Typescript:
type ThrottleFunction<T extends any[]> = (this: any, ...args: T) => any
function throttle<T extends any[]>(func: ThrottleFunction<T>, delay: number = 0): ThrottleFunction<T> {
let shouldThrottle = false
return function(...args){
if(shouldThrottle) return;
shouldThrottle = true // this will be true until delay time has passed.
setTimeout(function () {
shouldThrottle = false
}, delay)
func.apply(this, args) // alternatively, func.call(this, ...args)
}
}
The throttle function parameters are the same as the debounce function. In the function, first, we create a variable shouldThrottle
to decide whether the function be throttled or not. In the return function, we check if the throttle is active. If it is, we immediately return without invoking the callback function. After that, we set shouldThrottle
to true so subsequent calls will not invoke our callback function until the delay time has passed. Next, we set setTimeout
and after the given delay
time has passed, we set shouldThrottle
to false so, the next invocation will invoke the callback function. Finally, we invoke the callback function if shouldThrottle
is false.
Note that this article only covers the most common version of throttle to understand without worrying about other details that are not necessary to understand the idea behind the throttle function. There are other variations like Lodash’s “_.throttle” function.
If we look at Lodash’s implementation of the throttle
function, we can also see things like leading
and trailing
options and methods to flush
and cancel
the delayed callback function func
.
Another way of implementing the throttle
function is instead of ignoring all throttled function invocations when the state is active, we can collect all invocations and spread them out by executing them at every “delay” interval in the future while following the same rule that there can only be one invocation every delay
duration.
The throttle technique is not really ideal for autocomplete text boxes but when you’re dealing with things like drag and drop, resizing, scrolling or similar events that you need to update the value periodically then throttle could be an ideal use case.
Conclusion
If you are dealing with groups of events that need to be grouped together to save some money on server costs including bandwidth, data costs etc. And to make your application more performant overall, debounce and throttle are great ways to achieve this.